Void saveOrUpdate (Object object)throws HibernateException void saveOrUpdate (String entityName, Object object) throws HibernateException The saveOrUpdate method calls save or update method based on the operation. If the identifier exists, it will call update method else the save method will be called. So saveOrUpdate method calls save method if there is no record in database, and it calls update method if there is a record in database. Related Posts: getTransaction,beginTransaction,getIdentifier in Hibernate; CRUD operations using Hibernate+ Maven+ Oracle+ XML mapping. What is the difference between session.update(Object obj), session.merge(Object obj) and session.saveOrUpdate(Object obj) method of hibernate API? Session.update, session.merge and session.saveOrUpdate all three methods are used to update the records in database using hibernate persistence logic. The Session interface in Hibernate provides a couple of methods to move an object from a new or transient state to a persistent state e.g. Save, saveOrUpdate, and persist are used to store an object into the database, but there are some significant differences between them. The Session.save method does an INSERT to store the object into the database and it also returns.
Contents
- 6 How to prevent concurrent update in Hibernate?
Difference between session.save() , session.saveOrUpdate() and session.persist()?
session.save() : Save does an insert and will fail if the primary key is already persistent.session.saveOrUpdate() : saveOrUpdate does a select first to determine if it needs to do an insert or an update. Insert data if primary key not exist otherwise update data.
session.persist() : Does the same like session.save() and returns void.
Note: In case of using session.save() it returns Serializable object (i.e. primary key generated and stored in database for the concern Record).
System.out.println(session.save(question));
// This will print the generated primary key.
System.out.println(session.persist(question));
// Compile time error because session.persist() return void.
What is the difference between Hibernate and JDBC?
Hibernate | JDBC |
Hibernate is data base independent, your code will work for all ORACLE,MySQL ,SQLServer etc. | JDBC Queries are Database specific |
In Hibernate we use java objects as Tables to store in a database. One should only know Java syntax. | In case of JDBC we need to learn SQL Syntax. |
Query tuning is automatically handled by Hibernate. | We need to tune queries. |
Hibernate support two level of cache. First level and 2nd level. So you can store your data into Cache for better performance. | JDBC you need to implement your java cache |
Hibernate supports Query cache and It will provide the statistics about your query and database status | JDBC Not provides any statistics |
No need to create any connection pool in case of Hibernate. You can use c3p0. | In case of JDBC you need to write your own connection pool |
In the xml file you can see all the relations between tables in case of Hibernate. Easy readability | JDBC Don't have such support. |
You can load your objects on start up using lazy=false in case of Hibernate. | JDBC Don't have such support. |
Hibernate Supports automatic versioning of rows but JDBC Not. | JDBC Don't have such support. |
What is lazy fetching in Hibernate? Explain with example.
By default, Hibernate3 uses lazy select fetching for collections and lazy proxy fetching for single-valued associations. These defaults make sense for most associations in the majority of applications.If you set hibernate.default_batch_fetch_size, Hibernate will use the batch fetch optimization for lazy fetching. This optimization can also be enabled at a more granular level.
Please be aware that access to a lazy association outside of the context of an open Hibernate session will result in an exception. For example:
s = sessions.openSession();
Transaction tx = s.beginTransaction();
User u = (User) s.createQuery('from User u where u.name=:userName').setString('userName', userName).uniqueResult();
Map permissions = u.getPermissions();
tx.commit();
s.close();
Integer accessLevel = (Integer) permissions.get('accounts'); // Error!
This can be fixed by moving the code that reads from the collection to just before the transaction is committed.
Alternatively, you can use a non-lazy collection or association, by specifying lazy='false' for the association mapping. However, it is intended that lazy initialization be used for almost all collections and associations. If you define too many non-lazy associations in your object model, Hibernate will fetch the entire database into memory in every transaction.
On the other hand, you can use join fetching, which is non-lazy by nature, instead of select fetching in a particular transaction. We will now explain how to customize the fetching strategy.
In Hibernate3, the mechanisms for choosing a fetch strategy are identical for single-valued associations and collections.
what is the advantage of Hibernate over jdbc?
Advantages using Hibernate than JDBC:Difference Between Save Persist And Saveorupdate In Hibernate
- Hibernate is data base independent, your code will work for all ORACLE,MySQL ,SQLServer etc.In case of JDBC query must be data base specific.
- As Hibernate is set of Objects , you don?t need to learn SQL language. You can treat TABLE as a Object . Only Java knowledge is need.In case of JDBC you need to learn SQL.
- Don?t need Query tuning in case of Hibernate. If you use Criteria Quires in Hibernate then hibernate automatically tuned your query and return best result with performance. In case of JDBC you need to tune your queries.
- You will get benefit of Cache. Hibernate support two level of cache. First level and 2nd level. So you can store your data into Cache for better performance. In case of JDBC you need to implement your java cache .
- Hibernate supports Query cache and It will provide the statistics about your query and database status.JDBC Not provides any statistics.
- Development fast in case of Hibernate because you don?t need to write queries
- No need to create any connection pool in case of Hibernate. You can use c3p0. In case of JDBC you need to write your own connection pool
- In the xml file you can see all the relations between tables in case of Hibernate. Easy readability.
- You can load your objects on start up using lazy=false in case of Hibernate.JDBC Don?t have such support.
- Hibernate Supports automatic versioning of rows but JDBC Not.
How to Integrate Struts Spring Hibernate ?
TODO: SATISHHow to prevent concurrent update in Hibernate?
Using version checking in hibernate we can prevent concurent updates when more then one thread trying to access same data.For example :User A edit the row of the TABLE for update ( In the User Interface changing data - This is user thinking time) and in the same time User B edit the same record for update and click the update.Then User A click the Update and update done. Chnage made by user B is gone.
In hibernate you can perevent slate object updatation using version checking. Check the version of the row when you are upding the row. Get the version of the row when you are fetching the row of the TABLE for update. On the time of updation just fetch the version number and match with your version number ( on the time of fetching).This way you can prevent slate object updatation.
Difference Between Save And Saveorupdate In Hibernate With Example
Steps 1:Declare a variable 'versionId' in your Class with setter and getter.public class Campign {
private Long versionId;
private Long campignId;
private String name;
...
}
Step 2: edit.hbm.xml file
<class name='beans.Campign' table='CAMPIGN' optimistic-lock='version'>
<id name='campignId' type='long' column='cid'>
<generator>
<param name='sequence'>CAMPIGN_ID_SEQ</param>
</generator>
</id>
<version name='versionId' type='long' column='version' />
<property name='name' column='c_name'/>
</class>
Step 3: In the code
// foo is an instance loaded by a previous Session
session = sf.openSession();
int oldVersion = foo.getVersion();
session.load( foo, foo.getKey() );
if ( oldVersion!=foo.getVersion ) throw new StaleObjectStateException();
foo.setProperty('bar');
session.flush();
session.connection().commit();
session.close();
You can handle StaleObjectStateException() and do what ever you want.You can display error message.
Hibernate autumatically create/update the version number when you update/insert any row in the table.
Timestamp
The optional <timestamp> element indicates that the table contains timestamped data. This provides an alternative to versioning. Timestamps are a less safe implementation of optimisticlocking. However, sometimes the application might use the timestamps in other ways.How to perevent slate object updatation in Hibernate ?
TODO: SATISHWhat is version checking in Hibernate ?
TODO: SATISHHow to handle user think time using hibernate ?
TODO: SATISHTransaction with plain JDBC in Hibernate ?
If you don't have JTA and don't want to deploy it along with your application, you will usually have to fall back to JDBC transaction demarcation. Instead of calling the JDBC API you better use Hibernate's Transaction and the built-in session-per-request functionality:To enable the thread-bound strategy in your Hibernate configuration:
hibernate.transaction.factory_class = org.hibernate.transaction.JDBCTransactionFactory
hibernate.current_session_context_class = thread
Session session = factory.openSession();

Transaction tx = null;
try {
tx = session.beginTransaction();
// Do some work
session.load(...);
session.persist(...);
tx.commit(); // Flush happens automatically
}
catch (RuntimeException e) {
tx.rollback();
throw e; // or display error message
}
finally {
session.close();
}
What are the general considerations or best practices for defining your Hibernate persistent classes ?
- You must have a default no-argument constructor for your persistent classes and there should be getXXX() (i.e accessor/getter) and setXXX( i.e. mutator/setter) methods for all your persistable instance variables.
- You should implement the equals() and hashCode() methods based on your business key and it is important not to use the id field in your equals() and hashCode() definition if the id field is a surrogate key (i.e. Hibernate managed identifier). This is because the Hibernate only generates and sets the field when saving the object.
- It is recommended to implement the Serializable interface. This is potentially useful if you want to migrate around a multi-processor cluster.
- The persistent class should not be final because if it is final then lazy loading cannot be used by creating proxy objects.
Difference between session.update() and session.lock() in Hibernate ?
TODO: SATISHDifference between getCurrentSession() and openSession() in Hibernate ?
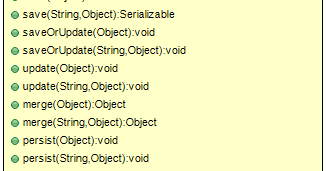
Difference between session.saveOrUpdate() and session.merge() ?
TODO: SATISHFilter in Hibernate with Example ?
TODO: SATISHHow does Value replacement in Message Resource Bundle work ?
TODO: SATISHDifference between list() and iterate() i9n Hibernate ?
TODO: SATISH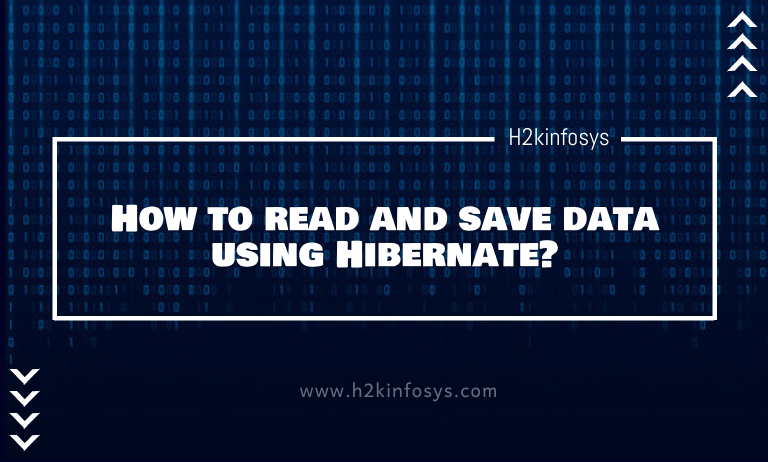
Difference between session.load() and session.get() ?
TODO: SATISHDeleting persistent objects
TODO: SATISHSQL statements execution order.
Difference Between Merge And Saveorupdate In Hibernate
TODO: SATISHDifference between session.saveOrUpdate() and session.merge() ?
TODO: SATISHModifying persistent objects ?
TODO: SATISHExplain SQL Queries In Hibernate?
TODO: SATISHFilter in Hibernate?
TODO: SATISHCriteria Query Two Condition
TODO: SATISHEqual and Not Equal criteria query.
TODO: SATISHCascade Save or Update in Hibernate ?
TODO: SATISHOne To Many Bi-directional Relation in Hibernate?
TODO: SATISHOne To Many Mapping Using List ?
TODO: SATISHMany To Many Relation In Hibernate ?
TODO: SATISHWhat does session.refresh() do ?
TODO: SATISHDifference between session.load() and session.get() ?
TODO: SATISHHibernate setup using .cfg.xml file ?
TODO: SATISHHow to add .hbm.xml file in sessionFactory ?
TODO: SATISHHow to get Hibernate statistics ?
TODO: SATISHHow to set 2nd level cache in hibernate with EHCache ?
TODO: SATISHHow to get JDBC connections in hibernate ?
TODO: SATISHHow will you configure Hibernate ?
TODO: SATISHHow to create Session and SessionFactory in Hibernate ?
TODO: SATISHWhat are the Instance states in Hibernate?
TODO: SATISHWhat are the core components in Hibernate ?
TODO: SATISHWhat is a Hibernate Session? Can you share a session object between different theads ?
TODO: SATISHWhat is the role of addScalar() method in hibernate?
TODO: SATISHHibernate session.close does _not_ call session.flush ?
TODO: SATISHWhat is the main difference between Entity Beans and Hibernate ?
TODO: SATISH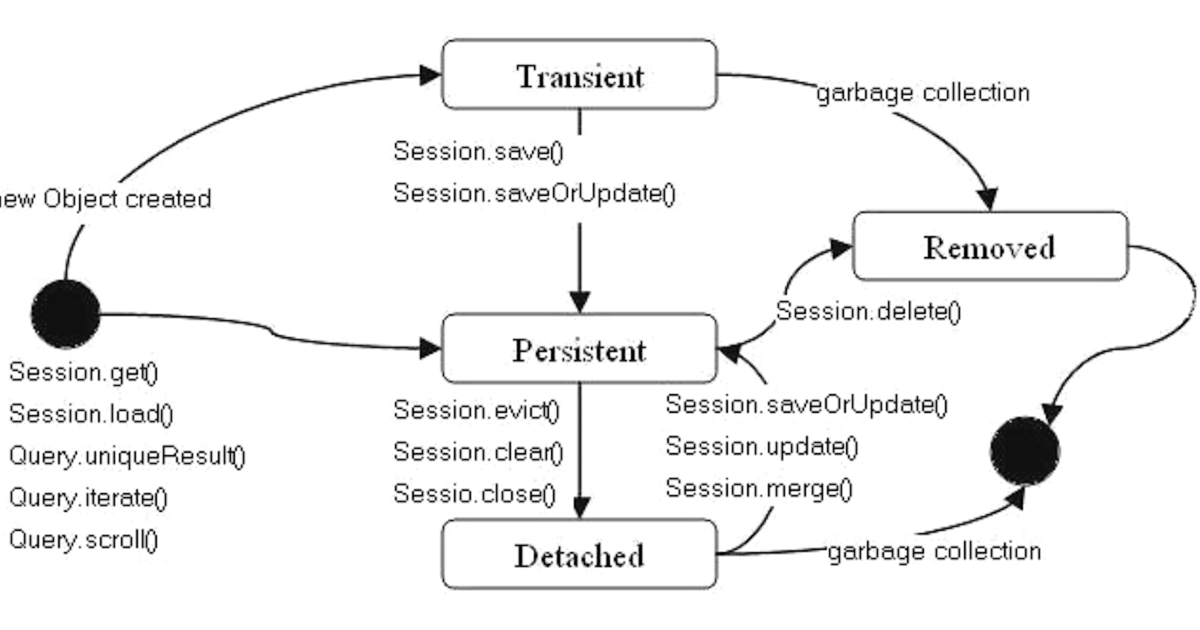
Update Vs Saveorupdate Hibernate
How are joins handled using Hinernate.
TODO: SATISH